日給で働く利点を理解し仕事を探す
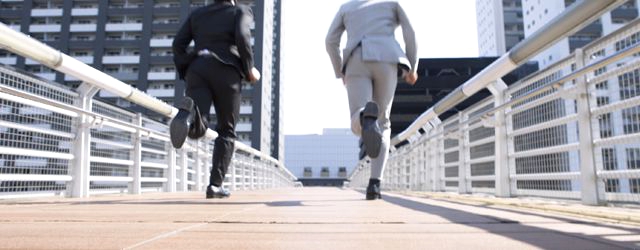
仕事探しの一つに、お給料をどの方法でもらうかを決めているならば、仕事の幅も広がります。お給料の制度の中には、日給制と時給制があることを知っておきましょう。これらの制度はパートやアルバイト、つまり、正社員以外の労働者に給料を支払う時に適用されます。日給で働くメリットの一つには、もらえる額のお給料が決まっているのでやりがいがあると言うことです。この制度で働く人たちは、建設現場や、警備など肉体労働に関わる人たちに適用されやすいお給料です。
この制度のお給料は、自分が出勤した日にはお給料は支払われますが、出勤しなかったり、何かのはずみで仕事がなかったりするとその分給料は発生しないと言うことがあるため、その点を覚えておく必要があります。例えば、建設などでは、雨がふれば行えないので、その日が何一も続けばその分はお給料にならないと言う設定です。また、祝日が多い月だと、その分仕事も減らされるので、そのことも念頭に置いておきましょう。お給料の支払い方は様々で、個人的にも、どの支払い方が良いのかと言う考えも様々です。自分のお仕事にたいする意欲が低下しないようなお給料のもらい方をして仕事を楽しめるように仕事を探しましょう。